Algorithm
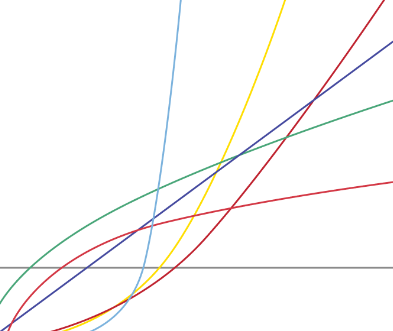
An algorithm is a clear sequence of actions, the implementation of which gives some kind of predetermined result. Simply put, it is a set of instructions for a specific task. This term is best known in computer science and computer science, where it is understood as instructions for solving a problem in an effective way.
Now this word is understood as any sequence of actions that can be clearly described and divided into simple steps and which lead to the achievement of a goal. For example, going to the kitchen, pouring water and putting a bag of tea in it is an algorithm for performing the "Brew Tea" task.
Algorithms in computer science are instructions for computers, a set of steps that are described by program code. There are specific algorithms for certain actions, and some of them are quite complex. One of the goals of using algorithms is to make the code more efficient and optimize it.
Who uses algorithms
In a general sense, absolutely all living and some non-living beings, because any sequence of actions leading to the goal can be considered an algorithm. The search for food by animals is an algorithm, the movements of the robot are also described by the algorithm.
In the narrow sense in which the concept is used in computer science, algorithms are used by developers, some engineers and analysts, as well as machine learning specialists, testers and many others. This is one of the key concepts in IT.
Why do we need algorithms?
Algorithms in computer science are needed to effectively solve various problems, including those whose execution "head-on" has a high complexity or is completely impossible. In practice, there are algorithms for almost anything: sorting, passing through data structures, searching for elements, filtering information, mathematical operations, and so on.
For example, you can sort an array in a brute-force search — this is the most obvious solution. And you can use the fast sorting algorithm: it is more complicated and not so obvious, but it works much faster and does not load the power of the computer so much. Strictly speaking, a complete search is also an algorithm, but very simple.
There are algorithmically unsolvable problems for which there is no algorithm and cannot exist. But most problems in IT are solvable algorithmically, and algorithms are actively used in working with them.
Algorithms are used in all areas of IT and in many other industries. Instructions for an automated machine or production line are algorithms, recipes are too. So in a general sense, algorithms are needed for anything.
Properties of algorithms
Discreteness. An algorithm is not a single indivisible structure, it consists of individual small steps, or actions. These actions go in a certain order, one begins after the completion of the other.
Effectiveness. Executing the algorithm should lead to some result and leave no uncertainty. The result may also be unsuccessful — for example, an algorithm may report that there is no solution — but it must be.
Determinism. At each step there should be no discrepancies and disagreements, the instructions should be clearly defined.
Massiveness. The algorithm can usually be extrapolated to similar problems with other source data - just change the initial conditions. For example, the standard algorithm for solving a quadratic equation will remain unchanged regardless of which numbers are used in this equation.
Intelligibility. The algorithm should include only actions that are known and understandable to the performer.
Limb. Algorithms are finite, they must complete and give the result, in some definitions - for a predetermined number of steps.
What are the algorithms
Despite the word "sequence," the algorithm does not always describe actions in a hard-coded order. This is especially true now, with the spread of asynchrony in programming. In algorithms, there is room for conditions, loops, and other nonlinear constructs.
Linear. This is the simplest type of algorithm: the actions follow each other, each begins after the previous one ends. They are not rearranged in places, are not repeated, are performed under any conditions.
Branching. In this type of algorithm, branching appears: some actions are performed only if some conditions are true. For example, if a number is less than zero, you must remove it from the data structure. You can also add a second branch: what to do if the condition is incorrect - for example, a number greater than zero or equal to it. There can be several conditions, they can be combined with each other.
Circular. Such algorithms are executed in a loop. When a block of actions ends, those actions start again and are repeated a number of times. A loop may include a single action or sequence, and the number of repetitions may be fixed or depend on a condition: for example, repeat this block of code until there are no empty cells left in the data structure. In some cases, the cycle can be infinite.
Recursive. Recursion is a phenomenon where some algorithm calls itself, but with different inputs. This is not a loop: the data is different, but there are several "instances" of running programs, not one. A well-known example of a recursive algorithm is the calculation of Fibonacci numbers.
Recursion allows you to elegantly solve some problems, but you need to be more careful with it: such algorithms can greatly load the resources of the system and work slower than others.
Probability. Such algorithms are mentioned less often, but this is a rather interesting type: the work of the algorithm depends not only on the input data, but also on random variables. These, for example, include the well-known algorithms of Las Vegas and Monte Carlo.
Basic and auxiliary. This is another type of classification. The main algorithm solves the immediate problem, the auxiliary one solves the subtask and can be used inside the main one - for this purpose, its name and input data are simply indicated there. An example of a helper algorithm is any software function.
Graphical representation of algorithms
Algorithms can write in text, code, pseudocode, or graphically as flowcharts. These are special schemes consisting of geometric shapes that describe certain actions. For example, the start and end points in the diagram are, respectively, the beginning and end of the algorithm, the parallelogram is the input or output of data, the rhombus is the condition. Simple actions are indicated by rectangles, and shapes are connected using arrows - they show sequences and cycles.
Algorithm complexity
The concept of "complexity" is one of the key in the study of algorithms. It does not mean how difficult it is to understand a particular method, but the resources spent on the calculation. If the complexity is high, the algorithm will run more slowly and possibly waste more hardware resources; it is desirable to avoid this.
Complexity is usually described by the large letter O. After it, the value on which the execution time depends is indicated in parentheses. It is a designation from mathematics that describes the behavior of different functions.
What a challenge. We will not fully analyze mathematical O-notation, as it is called, - we will simply list the main notations of complexity in the theory of algorithms.
- O(1) means that the algorithm is executed in a fixed constant time. These are the most efficient algorithms.
- O(n) is the complexity of linear algorithms. nhere and further denotes the size of the input data: the greaterthe n, the longer the algorithm runs.
- O(n²) also means that the greatern, the higher the complexity. But the dependence here is not linear, but quadratic, that is, the speed increases much faster. These are inefficient algorithms, for example, with nested loops.
- O(log n) is a more efficient algorithm. The speed of its execution is calculated logarithmically, that is, it depends on the logarithmn.
- O(√n) is an algorithm whose speed depends on the square root ofn. It is less efficient than logarithmic, but more efficient than linear.
There are also O(n³), O(nn) and other inefficient algorithms with high degrees. Their complexity grows very quickly, and it is better not to use them.
O-notation is used to assess whether to use a particular sequence of actions effectively. If the data is large or there are a lot of them, they try to look for more efficient algorithms to speed up the program.
Use of algorithms in IT
We will give some examples of the use of different algorithms in the programming industries. In fact, there are many more – we took only a part to help you understand the practical significance of algorithms.
Software and website development. Algorithms are used for parsing, that is, "parsing" structures with data, such as JSON. Parsing is one of the basic tasks, for example, in the web. Algorithms are also needed when drawing dynamic structures, displaying alerts, adjusting the behavior of the application and much more.
Work with data. Algorithms are very actively used when working with databases, files where information is stored, structures such as arrays or lists. There can be a lot of data, and choosing the right algorithm allows you to speed up the work with them. Algorithms solve the problems of sorting, changing and deleting the necessary elements, adding new data. With their help, they fill and pass through such structures as trees and graphs.
Algorithms are of particular importance in Big Data and data analysis: there they allow you to process a huge amount of information, including raw information, and not spend too many resources on it.
Search tasks. Search algorithms are a separate complex industry. They are allocated to a separate group, in which there are now dozens of different algorithms. Search is important in data science, in artificial intelligence methods, in analytics, and more. The most obvious example is search engines like Google or Yandex. By the way, the details about the algorithms used by search engines are usually kept secret.
Machine learning. In machine learning and artificial intelligence, the approach to algorithms is slightly different. If an ordinary program acts according to a given order of actions, then the "smart machine" - a neural network or a trained model - forms an algorithm for itself in the course of training. The developer describes the model and trains it: sets it the initial data and shows examples of what the final result should look like. In the course of training, the model itself thinks over an algorithm for achieving this result.
Such AI algorithms can be even more powerful than conventional ones and are used to solve problems that the developer is not able to break down into simple actions consciously. For example, to recognize objects, you need to use a huge number of processes in the nervous system: a person is simply physically unable to describe them all in order to repeat them programmatically.
During the creation and training of the model, the developer can also use algorithms. For example, the error propagation algorithm allows you to train neural networks.
- Arts
- Business
- Computers
- الألعاب
- Health
- الرئيسية
- Kids and Teens
- مال
- News
- Recreation
- Reference
- Regional
- Science
- Shopping
- Society
- Sports
- Бизнес
- Деньги
- Дом
- Досуг
- Здоровье
- Игры
- Искусство
- Источники информации
- Компьютеры
- Наука
- Новости и СМИ
- Общество
- Покупки
- Спорт
- Страны и регионы
- World