Understanding Computer Algorithms: The Heart of Modern Computing
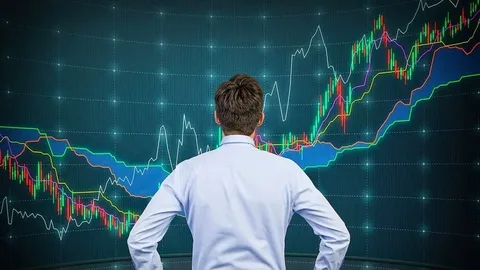
In the world of computer science, algorithms are fundamental building blocks that drive everything from simple software applications to complex machine learning models. But what exactly is an algorithm? Why are they so important? And how do they influence the performance and functionality of the systems we use every day?
What Is an Algorithm?
At its core, an algorithm is a step-by-step procedure or set of rules designed to perform a task or solve a problem. It takes an input, processes it, and produces an output. Think of it like a recipe: the ingredients (inputs) are transformed through a series of instructions (steps) to create the final dish (output).
For example, a simple algorithm to add two numbers would look something like this:
- Take two numbers as input.
- Add them together.
- Return the result.
It’s a clear, deterministic process. Algorithms can be incredibly simple (like the one above), or highly complex, depending on the task at hand.
Types of Algorithms
Algorithms are used in nearly every aspect of computing. Below are some of the major types:
-
Sorting Algorithms: Sorting algorithms arrange data in a specific order, often numerical or lexicographical. Common sorting algorithms include:
- Bubble Sort: A simple algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they’re in the wrong order.
- QuickSort: A divide-and-conquer algorithm that partitions the array into sub-arrays and recursively sorts them.
- MergeSort: Another divide-and-conquer approach that splits the array into halves, sorts each half, and then merges them back together.
-
Search Algorithms: Search algorithms help find specific data within a structure (e.g., an array or database). Examples include:
- Linear Search: Sequentially checks each element of a list until the target is found or the list ends.
- Binary Search: A more efficient algorithm for sorted lists, repeatedly dividing the search space in half to locate the target value.
-
Graph Algorithms: Graph algorithms operate on data structures known as graphs (sets of nodes connected by edges). These are critical in fields like networking, recommendation systems, and social media analysis. Notable examples are:
- Dijkstra’s Algorithm: Finds the shortest path between two nodes in a graph.
- Depth-First Search (DFS): Explores a graph by going as deep as possible along a branch before backtracking.
- Breadth-First Search (BFS): Explores all neighbors of a node before moving on to their neighbors.
-
Dynamic Programming Algorithms: Dynamic programming is used to solve problems by breaking them down into simpler subproblems and solving them just once, storing the results for future use. Famous examples include:
- Fibonacci Sequence: Efficient computation of Fibonacci numbers by storing intermediate results.
- Knapsack Problem: Solving optimization problems where you have to choose a subset of items with given weights and values that maximize profit without exceeding a weight limit.
-
Machine Learning Algorithms: In the domain of artificial intelligence and machine learning, algorithms are used to build models that can learn from data and make predictions. Some key algorithms include:
- Linear Regression: Predicts a dependent variable based on one or more independent variables.
- Decision Trees: A flowchart-like structure used to make decisions based on input data.
- K-means Clustering: Groups data into clusters based on similarity.
Algorithm Efficiency: Time and Space Complexity
One of the key concerns when designing algorithms is their efficiency. How quickly does an algorithm run (time complexity), and how much memory does it use (space complexity)? These are critical factors in determining how well an algorithm will scale as the size of the input grows.
Big O Notation is used to describe the upper bound of an algorithm’s complexity, helping developers understand how an algorithm will perform as the input size increases. Some common time complexities include:
- O(1): Constant time – The algorithm runs in the same time regardless of the input size.
- O(n): Linear time – The algorithm's time grows linearly with the input size.
- O(n^2): Quadratic time – The time grows proportionally to the square of the input size, common in algorithms with nested loops.
- O(log n): Logarithmic time – The algorithm's time grows logarithmically, which is very efficient for large inputs (e.g., binary search).
On the space complexity side, an algorithm's memory usage may grow with the input size. Optimizing both time and space is a central challenge when developing algorithms.
The Importance of Algorithms in Real Life
Algorithms are everywhere. In fact, they shape the way we interact with the world, often without us realizing it. Here are a few examples of how algorithms impact our daily lives:
-
Search Engines: Algorithms power search engines like Google, determining the most relevant search results based on your query. These algorithms consider factors like keyword relevance, page ranking, and user behavior.
-
Social Media Feeds: Platforms like Facebook and Instagram use algorithms to decide which posts, photos, or videos should appear in your feed, often based on your past interactions, likes, and time spent on the platform.
-
Recommendation Systems: Whether it’s Netflix recommending movies or Amazon suggesting products, algorithms analyze your past behavior and predict what you might like next.
-
Navigation Systems: Algorithms in GPS applications like Google Maps or Waze calculate the fastest routes based on real-time traffic data, road closures, and other factors.
-
Cybersecurity: Cryptographic algorithms are used to protect sensitive data, ensuring that your online transactions and personal information remain secure.
Conclusion: The Power and Potential of Algorithms
At its heart, an algorithm is a solution to a problem, and their importance can’t be overstated. They enable us to process and analyze vast amounts of data, automate tasks, and make complex systems run efficiently. With the rise of artificial intelligence, machine learning, and big data, algorithms are becoming increasingly sophisticated and crucial in driving innovation across industries.
Understanding how algorithms work, their complexities, and their potential for solving real-world problems is essential for anyone working in the tech industry or simply curious about the digital world. Whether you're coding a simple program or designing an AI-powered system, the principles of algorithms will be the guiding force behind your work.
- Arts
- Business
- Computers
- Jogos
- Health
- Início
- Kids and Teens
- Money
- News
- Recreation
- Reference
- Regional
- Science
- Shopping
- Society
- Sports
- Бизнес
- Деньги
- Дом
- Досуг
- Здоровье
- Игры
- Искусство
- Источники информации
- Компьютеры
- Наука
- Новости и СМИ
- Общество
- Покупки
- Спорт
- Страны и регионы
- World